



Processing the Withdrawal
In the last section of this tutorial, you created some variables to hold the
session bean's state, and implemented an SQL query that used information from
a database to fill in the state information. Now that you have the necessary
information in the bean, you can define behaviors that process the data in various
ways.
For this exercise, you will create a simple implementation of a transaction
that represents a bank withdrawal. The capsule needs to update both the information
it holds in its variables, and the information that should change in the database
as a result of the withdrawal. AppComposer's SQLUpdate actor allows you to modify
information in a database, just as the SQLSelect actor allowed you to retrieve
information from the database. Finally, you will implement some methods that
allow capsules or programs outside of the bean to access the state information
it holds.
You can summarize the steps for building this part of the capsule as follows:
- Updating the variables that hold the bean's state.
- Configuring an SQL query to update the database with the new state.
- Implementing capsule methods and behaviors that allow outside programs to
access the bean's state.
To implement the withdraw method:
- Create a new method on the capsule called withdraw.
- Select the capsule in the outline.
- Click the
icon next to Methods in the Details pane.
- Set the activation to withdraw.
- Set Transaction Level to Required.
- Add an argument called amount of type double.
- Click OK.
Since this method needs to commit information to a database, it should require
a transaction when it executes.
- Insert an action group as a child of the capsule and name it withdraw.
Set it to activate when it receives the withdraw method from the
capsule.

- You need to update the balance data item that holds the current
account balance. Insert an action behavior as a child of the withdraw
group and name it setBalance. This behaivor updates the balance
variable of the bean. The Eval field subtracts the amount
parameter from the value in balance, and sets that as the new value
for balance. Recall that the capsule method you defined includes
an argument called amount. This represents the amount of the withdrawal.
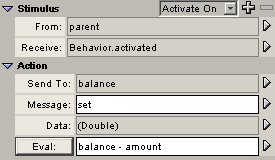
Notice that this behavior does not include error checking. Real beans should
always include this. For instance, you should be check to ensure that the
withdrawal amount is not more than the current balance.
So far you have a very simple implementation of a method to process a withdrawal
from an account and save the new state in the bean. Later you will add some
more behaviors to the withdraw group that update the information in
the database. Before you do that, you need to configure the SQLUpdate actor,
which determines what information in the database to change.
To configure the SQLUpdate actor:
- Add a SQLUpdate actor to the capsule and name it balanceUpdate.
- Click the Edit button in the details pane.
- In the Table tab, choose the ejbdata connection and ACCOUNTS
table as you did for the SQLSelect actor.
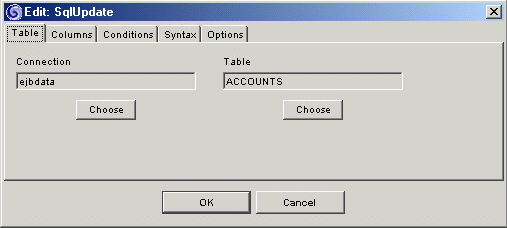
- In the Columns tab, update the BALANCE column with a parameter
(also) named BALANCE. The capsule feeds the query the value of this
parameter when the balanceUpdate actor executes.
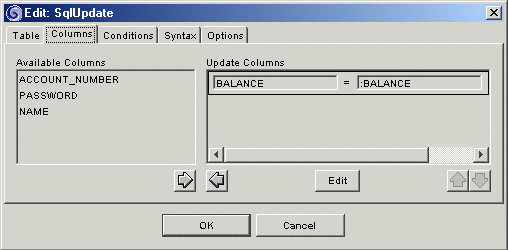
- In the Conditions tab, have it check that the column ACCOUNT_NUMBER
equals the value of the parameter called ACCOUNTNUM. This tells the
actor which account to update.
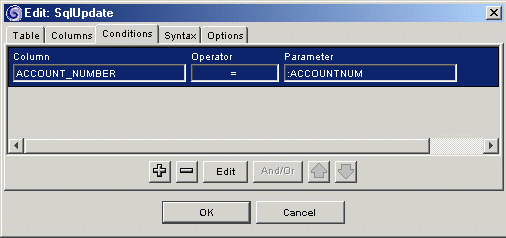
- Click OK.
Now that you have configured the update actor, you need to tell it when to
execute, and provide it with the parameters it needs to update the database.
Add some behaviors to the the withdraw action group so that it updates
the account in the database with the new balance.
To update the information in the database:
- As a child of the withdraw group, add an action and name it setBalanceParm.
Configure this so that it sets the balance parameter of the SQLUpdate
actor. Since you already calculated the new value of the capsule's balance
variable, use that to assign value to the SQLUpdate parameter with the same
name. Make sure that this behavior comes after the setBalance behavior
in the action group. When two behaviors activate on the same event, as these
two do, the capsule processes them in the order they appear in the outline.
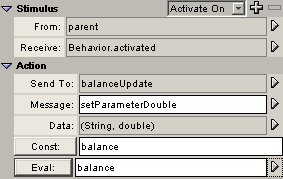
- As another child of withdraw, add an action behavior called setAccountParm.
Configure this behavior to send the accountNumber parameter that the bean
received with the create method to balanceUpdate's accountNum
variable.
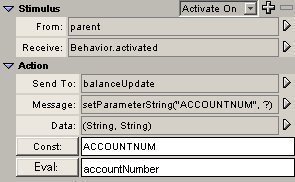
- Create an action to execute the database update. Name it execute update
and edit it so it sends a message of execute to balanceUpdate.
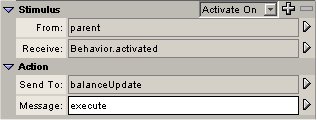
You have now implemented all of the business methods that use SQL. Your capsule
outline should look like this:
Sending out the Results
The session bean capsule needs two final business methods to be useful. Beyond
just updating the information in the bean and database, a client should be able
to request the current balance. The SimpleBank application needs to display
the customer's name and the balance of their account on a web page. The next
two behaviors you build access the state of the bean and do not change information
in the database. An outside capsule will act as a client and use these methods
through the finished session bean.
To retrieve the name:
- Create a new capsule method called getName that returns a String.
Leave the transactional attribute to the default, which is Supports.
This method does not need arguments.
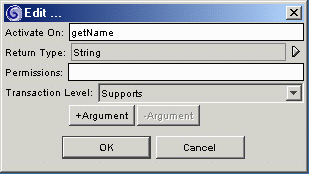
- Insert a return value behavior as a child of the capsule and name it returnName.
- The method should return the name variable when a client calls
the getName method.
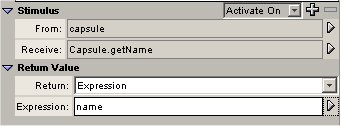
To retrieve the balance:
To implement the getBalance business method on the capsule, you need
a behavior that retrieves the balance value that was set in the ejbCreate
method.
- Create a new capsule method called getBalance that returns a double.
Leave the transactional attribute to the default, which is Supports.
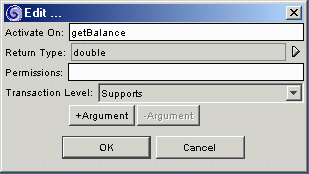
- Insert a return value behavior as a child of the capsule and name it returnBalance.
- Set the behavior to return the balance attribute when the client
calls the getBalance method.
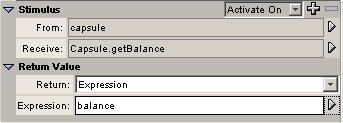
If you are familiar with EJBs, you may be interested in what the source code
of your bean looks like at this point. Select Source from the Capsule
menu. You see the implementation of the getBalance and getName
methods in the bean implementation and in the remote interface. Notice that
the methods in the remote interface throw a RemoteException, as required by
the EJB specification.
You have built a functionally complete session bean capsule. It can get information
from and update a database, and send that information out on request. At this
point, your capsule outline should look like this:
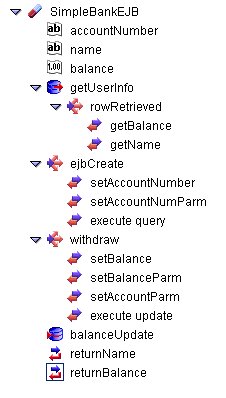
You are ready to generate, deploy and use you session bean. The next
section of the tutorial shows you how to finish off and test your session
bean capsule.




© DigiSlice 2003